반응형
Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 스프링
- spring
- VUE
- 국비IT
- 자바스크립트
- Java의정석
- CSS
- 리액트네이티브
- 평일코딩
- typescript
- ReactNative
- react
- 오라클
- 정보처리기사실기요약
- 국비코딩
- 정보처리기사
- 자바스크립트 코딩테스트
- 리액트
- 정보처리기사실기정리
- php
- 자바의정석
- 자스코테
- 정보처리기사요약
- 정보처리기사정리
- Oracle
- 코딩테스트
- 이안의평일코딩
- 정보처리기사실기
- javascript
- 타입스크립트
Archives
- Today
- Total
이안의 평일코딩
[PHP] POST방식과 CRUD (rename, unlink) 본문
반응형
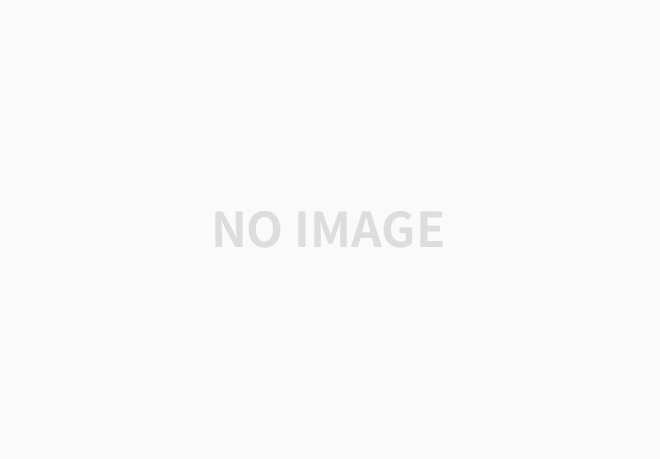
form과 POST
post로 데이터를 보내면 post로 받아야하고 get으로 보내면 get으로 받는다.
get은 url에 보이지만 post는 노출되지 않고 보낼 수 있다.
(method를 생략하면 기본으로 get방식이 적용된다. 북마크용도로는 get이 좋지만 CRUD에는 post를 사용)
form.html
<!DOCTYPE html>
<html>
<body>
<form action="form.php" method="post">
<p><input type="text" name="title" placeholder="Title"></p>
<p><textarea name="description" placeholder="Description"></textarea></p>
<p><input type="submit"></p>
</form>
</body>
</html>
form.php
<?php
file_put_contents('data/'.$_POST['title'], $_POST['description']);
?>
CREATE
file_put_contents(파일이 생성될 경로 제목, 파일 내용)
header: redirection될 경로
create.php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>
<?php
print_title();
?>
</title>
</head>
<body>
<h1><a href="index.php">WEB</a></h1>
<ol>
<?php
print_list();
?>
</ol>
<a href="create.php">create</a>
<form action="create_process.php" method="post">
<p>
<input type="text" name="title" placeholder="Title">
</p>
<p>
<textarea name="description" placeholder="Description"></textarea>
</p>
<p>
<input type="submit">
</p>
</form>
</body>
</html>
create_process.php
<?php
file_put_contents('data/'.$_POST['title'], $_POST['description']);
header('Location: /index.php?id='.$_POST['title']);
?>
UPDATE
if(isset())을 이용하여 메인화면이 아닌 id가 존재하는 화면에서만 update가 보이게 조건을 건다.
index.php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>
<?php
print_title();
?>
</title>
</head>
<body>
<h1><a href="index.php">WEB</a></h1>
<ol>
<?php
print_list();
?>
</ol>
<a href="create.php">create</a>
<?php if(isset($_GET['id'])) { ?>
<a href="update.php?id=<?=$_GET['id'];?>">update</a>
<?php } ?>
<h2>
<?php
print_title();
?>
</h2>
<?php
print_description();
?>
</body>
</html>
url 파라미터 id값: $_GET['id']
<?php if(isset($_GET['id'])) { ?>
<a href="update.php?id=<?php echo $_GET['id']; ?>">update</a>
<?php } ?>
<?php echo ?>는 <?= ?>로 수정이 가능하다.
<?php if(isset($_GET['id'])) { ?>
<a href="update.php?id=<?=$_GET['id']; ?>">update</a>
<?php } ?>
input에서 value라는 속성을 이용하면 수정할 원래 값(기본 값)을 채울 수 있다.
그리고 hidden이라는 속성을 이용하면 사용자에게 감춰 서버로만 값을 보낼 수 있다.
update.php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>
<?php
print_title();
?>
</title>
</head>
<body>
<h1><a href="index.php">WEB</a></h1>
<ol>
<?php
print_list();
?>
</ol>
<a href="create.php">create</a>
<?php if(isset($_GET['id'])) { ?>
<a href="update.php?id=<?=$_GET['id'];?>">update</a>
<?php } ?>
<form action="update_process.php" method="post">
<input type="hidden" name="old_title" value="<?=$_GET['id']?>">
<p>
<input type="text" name="title" placeholder="Title" value="<?php print_title(); ?>">
</p>
<p>
<textarea name="description" placeholder="Description"><?php print_description(); ?></textarea>
</p>
<p>
<input type="submit">
</p>
</form>
</body>
</html>
rename(수정전이름, 수정후이름)
file_put_contents(생성 파일 제목, 파일 내용)
update_process.php
<?php
rename('data/'.$_POST['old_title'], 'data/'.$_POST['title']);
file_put_contents('data/'.$_POST['title'], $_POST['description']);
header('Location: /index.php?id='.$_POST['title']);
?>
DELETE
delete는 form거칠 필요가 없기 때문에 바로 delete_process.php링크로 한다.
하지만 a 링크를 통해 삭제가 된다면 공유한 링크를 클릭하는 것만으로도 삭제가 되거나 하기 때문에 form으로 만든다.
<a href="delete_process.php?id=<?=$_GET['id'];?>">delete</a>
post방식으로 숨겨서 id는 input의 hidden 속성으로 넘겨준다.
index.php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>
<?php
print_title();
?>
</title>
</head>
<body>
<h1><a href="index.php">WEB</a></h1>
<ol>
<?php
print_list();
?>
</ol>
<a href="create.php">create</a>
<?php if(isset($_GET['id'])) { ?>
<a href="update.php?id=<?=$_GET['id'];?>">update</a>
<form action="delete_process.php" method="post">
<input type="hidden" name="id" value="<?=$_GET['id']?>">
<input type="submit" value="delete">
</form>
<?php } ?>
<h2>
<?php
print_title();
?>
</h2>
<?php
print_description();
?>
</body>
</html>
unlink(삭제할 파일);
delete_process.php
<?php
unlink('data/'.$_POST['id']);
header('Location: /index.php')
?>
반응형
'Back-end > PHP' 카테고리의 다른 글
[PHP] 리팩토링 (require), XSS, htmlspecialchars, basename (0) | 2021.04.02 |
---|---|
[PHP] 함수의 활용 (return) (0) | 2021.04.02 |
[PHP] 반복문과 조건문의 활용 (scandir) (0) | 2021.04.02 |
[PHP] PHP의 기초(2) - 함수, 조건문, 반복문, 배열 (0) | 2021.04.02 |
[PHP] PHP의 기초(1) - 환경설정, 데이터타입, 변수, 파라미터 (0) | 2021.04.01 |