일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 리액트
- ReactNative
- react
- 정보처리기사요약
- 평일코딩
- 자바의정석
- 자바스크립트 코딩테스트
- 국비코딩
- 정보처리기사실기
- VUE
- php
- spring
- 자바스크립트
- 정보처리기사실기요약
- 정보처리기사정리
- Oracle
- 오라클
- 이안의평일코딩
- 타입스크립트
- 정보처리기사실기정리
- 스프링
- 정보처리기사
- typescript
- javascript
- 자스코테
- 국비IT
- 코딩테스트
- Java의정석
- 리액트네이티브
- CSS
- Today
- Total
이안의 평일코딩
JAVA의 정석 16일차 - 메소드 본문
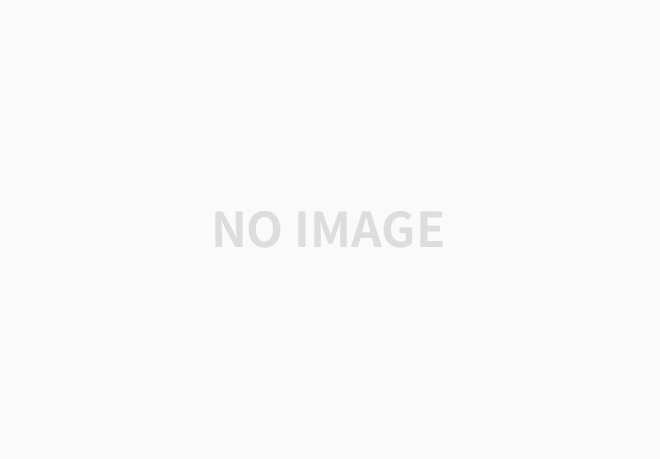
2020.07.06(월)
1. 메소드
1) 자동으로 메모리에 저장 => 정적 메소드
=>
static 리턴형(결과값) 메소드명(매개변수...) {
return 값
}
2) 프로그래머가 메모리를 만든 다음에 저장 => 인스턴스 메소드
===> new연산자를 이용하면 저장이 가능
=> 프로그램의 중심
리턴형(결과값) 메소드명(매개변수..) {
return 값
}
3) 메소드의 선언부만 사용 => 추상 메소드
=>
리턴형(결과값) 메소드명(매개면수...); => 추상 클래스(인터페이스)
2. 메소드 형태
1) 선언부 => 메모리에 저장이 안된다
2) 구현부 => 메모리에 저장 => 필요한 위치에서 사용이 가능
리턴형 메소드명(매개변수...) => 선언부
구현부 {
가공 (처리)
return 결과값
}
결과값이 없는 경우에는 return을 생략이 가능
=> JVM => 자동으로 return을 추가
return : 메소드가 종료하는 시점 ==> 모든 메소드에는 return이 존재
=> 리턴형(결과값) ===> 결과값은 반드시 한개만 설정
기본형 (정수, 실수...)
데이터가 여러개일 경우 => 데이터를 모아서 한번에 전송(배열, 클래스)
=> 사용자 입력값(매개변수, 인자, 인수) ==> 사용자 요청값
한개일 수 있고
여러개 일 경우도 있다 ==> 매개변수는 3개 이상 초과(X)
====== 배열, 클래스
=> 10명의 국어점수 => 평균
(int a1,.... int a10) => (int[] kor)
=> return문 : 모든 메소드에서 반드시 사용 => 메소드 종료
위치는 상관없다
예외) 결과값이 없는 경우에는 생략가능
결과값?(리턴값, 반환값)
사용자로부터 받는 값?(매개변수,인자,인수...)
사용자로부터 정수를 입력 받아서 => 해당 구구단을 출력
1) 결과값(O), 매개변수(O) => 구구단을 묶어서 넘겨준다
String
static String gugudan1(int dan) {
// 출력
return "";
}
import java.util.*;
public class 자바메소드1 {
static String gugudan1(int dan) {
String gu="";
for(int i=1; i<=9; i++) {
gu+=dan+"*"+i+"="+dan*i+"\n";
}
return gu;
}
public static void main(String[] args) {
String gu = gugudan1(9); // 메소드 수행후에 결과값을 넘겨주고
// 다음문장으로 넘어간다
System.out.println(gu);
return; // 메소드 종료
}
}
for(int i=2; i<=9; i++) {
String gu=gugudan1(i); // 반복적인 기능의 제거
System.out.println(gu);
}
2) 결과값(X), 매개변수(O) => 메소드안에서 출력 (***)
void
static void gugudan2(int dan) {
// 전체 처리 ==> 출력
for(int i=1; i<=9; i++ {
System.out.printf("%d*%d=%d\n",dan,i,dan*i);
}
}
* 구구단 출력
import java.util.*;
public class 자바메소드1 {
static void gugudan2(int dan) {
for(int i=1; i<=9; i++) {
System.out.printf("%d*%d=%d\n",dan,i,dan*i);
}
}
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.print("정수 입력>");
int dan = scan.nextInt();
// 구구단을 출력할 메소드 호출
gugudan2(dan); // 입력한 단 구구단 출력
// 메소드는 호출하면 => 항상 메소드의 시작부터 종료까지 반복해서 출력
gugudan2(3); // 3단 출력
gugudan2(5); // 5단 출력
String gu = gugudan1(9); // 메소드 수행후에 결과값을 넘겨주고
// 다음문장으로 넘어간다
// System.out.println(gu);
for(int i=2; i<=9; i++) {
String gu = gugudan1(i); // 반복적인 기능의 제거
System.out.println(gu);
}
return; // 메소드의 종료 ==> 자동 추가 // void는 생략가능
}
}
* Genie뮤직 탑50 데이터 긁어오기 (라이브러리 jsoup-1.13.1jar 활용)
import java.util.*;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.select.Elements;
/*
* 예외처리 => 에러방지
* => 에러 => 직접처리 => try~catch
* => 에러 => 회피(시스템에 맡긴다) => throws(안쓰면 오류남)
* <td class="info">
<a href="#" class="title ellipsis" title="재생" onclick="fnPlaySong('90443299','1');return false;">
How You Like That</a>
*/
public class 자바메소드2 {
static String[] genieMusicData() throws Exception {
String[] data = new String[50];
Document doc = Jsoup.connect("https://www.genie.co.kr/chart/top200").get();
org.jsoup.select.Elements title = doc.select("td.info a.title");
for(int i=0; i<50; i++) {
data[i]=title.get(i).text();
}
return data;
}
public static void main(String[] args) throws Exception {
String[] data = genieMusicData();
int count = 1;
for(String title:data) {
System.out.println(count+"위 "+title);
count++;
}
}
}
* Genie뮤직 탑50 데이터 검색
=> 곡명을 입력하세요:보라
==> 보라빛 밤 (pporappippam)
import java.util.*;
import javax.lang.model.util.Elements;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
/*
* <td class="info">
<a href="#" class="title ellipsis" title="재생" onclick="fnPlaySong('90443299','1');return false;">
How You Like That</a>
*/
public class 자바메소드2 {
static String[] genieMusicData() throws Exception {
String[] data = new String[50];
Document doc = Jsoup.connect("https://www.genie.co.kr/chart/top200").get();
org.jsoup.select.Elements title = doc.select("td.info a.title");
for(int i=0; i<50; i++) {
data[i]=title.get(i).text();
}
return data;
}
static String[] genieMusicFind(String searchString) throws Exception {
String[] data=genieMusicData();
int k=0;
for(String title:data) {
if(title.contains(searchString)) {
k++;
}
}
String[] find = new String[k];
k=0;
for(String title:data) {
if(title.contains(searchString)) {
find[k]=title;
k++;
}
}
return find;
}
public static void main(String[] args) throws Exception {
String[] data = genieMusicData();
int count = 1;
for(String title:data) {
System.out.println(count+"위 "+title);
count++;
}
System.out.println("==========================");
Scanner scan = new Scanner(System.in);
System.out.print("곡명을 입력하세요:");
String searchString = scan.nextLine();
String[] fd=genieMusicFind(searchString);
for(String title:fd) {
System.out.println(title);
}
}
}
3. 메소드 호출
프로그램 (저장된 데이터 + 명령문)
변수 ==> 배열 ==> 클래스 ==> 파일 ==> 데이터베이스
============
명령문(;세미콜론) => 메소드 (한개의 기능, 재사용) => 클래스 => 패키지 => .jar(라이브러리)
=========
메소드
= 사용자 정의 메소드
= 형식)
반환형 메소드명(매개변수...) {
return 값; => 값=반환형
반환형이 없는 경우에는 => void, return은 생략이 가능
}
==> 메소드안에서 처리 => 출력
===> 일반 도스
==> 메소드안에서 처리 => 결과값을 전송할 수 있다
===> 웹, 윈도우
= 호출)
메소드를 호출할때는
메소드명(값,값)
=> void method(int a, int b)
=> method(10,20)
메소드를 호출하고 => 메소드가 수행이 종료(return)
=> 다시 호출된 위치로 돌아 온다
=> int method()
=> 호출
int a = method()
=> String method(int a)
=> 호출
String s = method(10)
=> double method(int a)
=> 호출
double d = method(10) => int a =10
=> double random()
=> String substring(int s, int e)
=> boolean equals(String s)
=> void println()
반환값(리턴형) | 매개변수 | |
O | O | String substring(int s, int e) |
O | X | int length() double random() |
X (void) | O | System.out.println(String s) |
X (void) | X | System.out.println() => 다음줄에 출력 |
void = 실행결과가 없음, 돌려줄 값이 없음. 출력까지 처리.
= 라이브러리 메소드
============== +
메소드: 특정 작업을 수행하기 위한 컴퓨터의 명령문의 집합
==> *** 한가지 일만 수행
*** 세분화 작업
======== 재사용의 목적 => import
목적 : 재사용성, 중복된 코드 제거
사용법 : 구조화 (단락(기능))
예) 숫자 야구 게임
= 3자리 정수의 난수 발생
= 사용자에게 입력을 요청
= 난수와 사용자 입력값을 비교
= 힌트
= 정답일 경우에 종료
= 다시 게임을 할지 여부 확인
실행 형식 => 메소드가 종료해야 => 다른 문장을 수행한다
1) 목록 출력
2) 찾기(검색)
3) 수정
4) 삭제
5) 추가
6) 상세보기
====> 판매
(1)
public class 자바메소드1 {
static void aaa()
{
System.out.println("aaa() 호출 시작...");//1
bbb();// bbb() 이동
System.out.println("aaa() 호출 종료...");//8
}
static void bbb()
{
System.out.println("bbb() 호출 시작...");//2
ccc();// ccc() 이동
System.out.println("bbb() 호출 종료...");//7
}
static void ccc()
{
System.out.println("ccc() 호출 시작...");//3
ddd();// ddd() 이동
System.out.println("ccc() 호출 종료...");//6
}
static void ddd()
{
System.out.println("ddd() 호출 시작...");// 4
System.out.println("ddd() 호출 종료...");// 5
}
public static void main(String[] args) {
aaa();// 시작하는 메소드를 호출 (시작점만 호출)
}
}
(2) 사칙연산 처리
import java.util.*;
public class 자바메소드2 {
static int plus(int a,int b)
{
return a+b;
}
static int minus(int a,int b)
{
return a-b;
}
static int gob(int a,int b)
{
return a*b;
}
static void div(int a,int b)
{
if(b==0)
System.out.println("0으로 나눌 수 없습니다");
else
System.out.printf("%d / %d = %.2f\n",a,b,a/(double)b);
return;
}
public static void main(String[] args) {
// 사용자 요청값 ==> 정수 , 정수
Scanner scan=new Scanner(System.in);
System.out.print("정수 입력(5 2):");
int a=scan.nextInt();
int b=scan.nextInt();
System.out.print("연산자 입력(+,-,*,/):");
String op=scan.next();
int result=0;
// 메소드 호출
switch(op)
{
case "+":
result=plus(a,b);
System.out.printf("%d + %d = %d\n",a,b,result);
break;
case "-":
result=minus(a,b);
System.out.printf("%d - %d = %d\n",a,b,result);
break;
case "*":
result=gob(a,b);
System.out.printf("%d * %d = %d\n",a,b,result);
break;
case "/":
div(a,b);
break;
default:
System.out.println("잘못된 연산자입니다!!");
}
}
}
(3)
public class 자바메소드3 {
/*
* static int plus(int a,int b) { return a+b; }
*/
public static void main(String[] args) {
int a=10;
int b=20;
// 재사용성
int result=자바메소드2.plus(a, b); //같은 폴더에 있으면 import 안써도됨.
System.out.println(result);
}
}
(4)
1) 데이터 10개(영화제목)를 가지고 오는 메소드의 원형을 작성하시오
=> String[] movieList();
2) 사용자가 ID, PWD를 입력하면 => 로그인 (true), 실패 (false)
=> boolean login(String id, String pwd)
3) 영화 번호를 넘겨주면 => 장르를 넘겨주는 메소드
=> String getGenre(int no)
4) 검색을 하면 찾은 영화명을 넘겨주는 메소드 제작
=> String movieFind(String ss)
5) 영화 예매 상위 5개를 넘겨주는 메소드 제작
=> String[] pop5() // 1개이상이면 배열로 잡아줌
==> 구현은 나중
==> 원형
1) 반환값
2) 사용자가 보내주는 데이터
예1) ID 중복체크
==> 데이터변환타입 boolean 메서드이름 idCheck 매개변수 (String id)
boolean idCheck(String id)
예2) 우편번호 찾기
==> 여러개나옴 배열 String[] postFind(String dong)
예3) 검색 => 검색된 내용을 출력
==> String[] find(String ss)
예4) 리뷰
==> String[] review(String re)
//매개변수는 갯수 제한이 없음.
//메소드 : 사용자가 요청한 값 (갯수)
요청 처리 => 결과값은 ?
==> 구현
import java.io.*;
import java.net.*;
public class 자바메소드4 {
static void getData() throws Exception
{
URL url=new URL("https://www.daum.net");
HttpURLConnection conn=(HttpURLConnection)url.openConnection();
if(conn!=null)
{
BufferedReader in=
new BufferedReader(new InputStreamReader(conn.getInputStream(),"UTF-8"));
while(true)
{
String str=in.readLine();
if(str==null)break;
System.out.println(str);
}
}
}
public static void main(String[] args) throws Exception{
getData();
}
}
4. 메소드 응용
1) 메소드 : 재사용 목적 (사용이 편리하게 제작)
반복 제거 (밑 예시의 오라클연결,닫기 반복된 부분을 메소드로 처리하면 편리하게 쓸 수 있다)
select() {
오라클 연결
select문장 수행
오라클 닫기
}
delete() {
오라클 연결
delete문장 수행
오라클 닫기
}
update() {
오라클 연결
update문장 수행
오라클 닫기
}
find() {
오라클 연결
find문장 수행
오라클 닫기
}
inser() {
오라클 연결
insert문장 수행
오라클 닫기
}
2) 연습문제
5개의 정수를 보낸다
5개 정수 => SORT => 전송
====
select
public class 자바메소드1 {
static int[] sort(int[] arr, String type) {
// sort
for(int i=0; i<arr.length-1; i++) {
for(int j=i+1; j<arr.length; j++) {
if(type.equals("ASC")){
if(arr[i]>arr[j]) { // 오름차순
int temp = arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
if(type.equals("DESC")){ //내림차순
if(arr[i]<arr[j]) {
int temp = arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
}
}
return arr;
}
public static void main(String[] args) {
// 호출
int[] arr = {10,40,30,50,20};
System.out.println("정렬 전:");
for(int i:arr) {
System.out.print(i+" ");
}
System.out.println("\n정렬 후:");
int[] result = sort(arr, "ASC"); //sort =>10,20,30,40,50, "DESC"는 반대
for(int i:result) {
System.out.print(i+" ");
}
}
}
public class 자바메소드1 {
// 내림차순의 메소드
static char[] sort(char[] arr,String type)
{
// sort
for(int i=0;i<arr.length-1;i++)
{
for(int j=i+1;j<arr.length;j++)
{
if(type.equals("ASC"))
{
if(arr[i]>arr[j])
{
char temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
if(type.equals("DESC"))
{
if(arr[i]<arr[j])
{
char temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
}
}
return arr;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
// 호출
char[] arr={'A','B','D','E','C'}; // sort => 10,20,30,40,50
System.out.println("정렬 전:");
for(char i:arr)
{
System.out.print(i+" ");
}
System.out.println("\n정렬 후:");
/*
* for(int i=0;i<arr.length-1;i++)
{
for(int j=i+1;j<arr.length;j++)
{
if(type.equals("ASC"))
{
if(arr[i]>arr[j])
{
char temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
}
}
*/
char[] result=sort(arr,"ASC");
for(char i:result)
{
System.out.print(i+" ");
}
System.out.println();
/*
* for(int i=0;i<arr.length-1;i++)
{
for(int j=i+1;j<arr.length;j++)
{
if(type.equals("DESC"))
{
if(arr[i]<arr[j])
{
char temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
}
}
Image dimg = img.getScaledInstance(label.getWidth(), label.getHeight(),
Image.SCALE_SMOOTH);
*/
result=sort(arr,"DESC");
for(char i:result)
{
System.out.print(i+" ");
}
}
}
public class RecipeVO {
private int no;
private String title;
private String poster;
private String chef;
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getPoster() {
return poster;
}
public void setPoster(String poster) {
this.poster = poster;
}
public String getChef() {
return chef;
}
public void setChef(String chef) {
this.chef = chef;
}
}
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.select.Elements;
/*
* <div class="col-xs-3">
<a class="thumbnail" href="/recipe/6924266">
<span class="thumbnail_over"><img src="https://recipe1.ezmember.co.kr/img/thumb_over.png"></span>
<img src="https://recipe1.ezmember.co.kr/cache/recipe/2019/12/30/77b62c185a190a93c86dac00fe51e0d81_m.jpg" style="width:275px; height:275px;">
<div class="caption">
<h4 class="ellipsis_title2">밥솥 오리고기 짜장라면(몸에 ...</h4>
<p>by 밥솥개미</p>
</div>
</a>
<div style="position:absolute;top:365px;width:100%;text-align:right;right:20px;"><span style="color:#74b243;font-size:10px;" class="glyphicon glyphicon-certificate"></span></div>
</div>
*/
public class RecipeManager {
public static void main(String[] args) {
RecipeManager rm=new RecipeManager();
rm.recipeListData();
}
public static RecipeVO[] recipeListData()
{
RecipeVO[] data=new RecipeVO[40];
try
{
Document doc=Jsoup.connect("https://www.10000recipe.com/recipe/list.html").get();
Elements title=doc.select("div.caption h4.ellipsis_title2");
Elements poster=doc.select("a.thumbnail img[src*=/recipe/]");
Elements chef=doc.select("div.caption p");
for(int i=0;i<title.size();i++)
{
System.out.println(title.get(i).text());
System.out.println(poster.get(i).attr("src"));
System.out.println(chef.get(i).text());
System.out.println("==========================");
RecipeVO vo=new RecipeVO();
vo.setNo(i+1);
vo.setChef(chef.get(i).text());
vo.setTitle(title.get(i).text());
vo.setPoster(poster.get(i).attr("src"));
data[i]=vo;
}
}catch(Exception ex) {}
return data;
}
}
import java.util.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.net.URL;
public class RecipeMain extends JFrame{
JPanel pan=new JPanel();
public RecipeMain()
{
try
{
pan.setLayout(new GridLayout(5,4,5,5));
RecipeVO[] r=RecipeManager.recipeListData();
int k=0;
for(RecipeVO rv:r)
{
if(k>19) break;
System.out.println(rv.getChef());
pan.add(new JLabel(new ImageIcon(getImage(new ImageIcon(new URL(rv.getPoster())),250,200))));
k++;
}
add("Center",pan);
setSize(1024, 800);
setVisible(true);
}catch(Exception ex) {ex.printStackTrace();}
}
public Image getImage(ImageIcon ii,int w,int h)
{
Image dimg = ii.getImage().getScaledInstance(w, h,
Image.SCALE_SMOOTH);
return dimg;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
try
{
UIManager.setLookAndFeel("com.jtattoo.plaf.mcwin.McWinLookAndFeel");
}catch(Exception ex) {ex.printStackTrace();}
new RecipeMain();
}
}
'Back-end > Java' 카테고리의 다른 글
JAVA의 정석 18일차 - 멤버변수와 공유변수 (0) | 2020.07.08 |
---|---|
JAVA의 정석 17일차 - 메소드 응용 (0) | 2020.07.07 |
JAVA의 정석 15일차 - 변수, 연산자, 제어문, 배열, 문자열 복습 (0) | 2020.07.03 |
JAVA의 정석 14일차 - 배열용도2 (0) | 2020.07.02 |
JAVA의 정석 13일차 - 배열용도 (0) | 2020.07.01 |