반응형
Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 이안의평일코딩
- 자스코테
- 리액트네이티브
- Java의정석
- VUE
- 국비코딩
- Oracle
- 평일코딩
- spring
- 국비IT
- 타입스크립트
- 자바의정석
- CSS
- 코딩테스트
- 오라클
- php
- 스프링
- 정보처리기사실기요약
- react
- 정보처리기사실기정리
- 정보처리기사정리
- 자바스크립트 코딩테스트
- 리액트
- typescript
- 자바스크립트
- 정보처리기사요약
- ReactNative
- 정보처리기사실기
- 정보처리기사
- javascript
Archives
- Today
- Total
이안의 평일코딩
Spring 11일차 - React 본문
반응형
2020.11.11(수)
Service => 사용자 요청을 받아서 데이터베이스 연동 => JSP로 결과값 전송 (Model)
MVC구조
- Model (java) => Service (Spring : Controller 요청을 제어하는 프로그램)
- View (jsp) => 화면 출력 (Spring : JSP)
- Controller => Model+JSP (Spring : DispatcherServlet : Front Controller)
요청을 받아서 Model+JSP를 연결해주는 역할
SpringSubqueryProject
src/main/java
com.sist.dao
EmpVO
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
|
package com.sist.dao;
import java.util.*;
public class EmpVO {
private int empno;
private String ename;
private String job;
private Date hiredate;
private int sal;
private int deptno;
private String dname;
private String loc;
public int getEmpno() {
return empno;
}
public void setEmpno(int empno) {
this.empno = empno;
}
public String getEname() {
return ename;
}
public void setEname(String ename) {
this.ename = ename;
}
public String getJob() {
return job;
}
public void setJob(String job) {
this.job = job;
}
public Date getHiredate() {
return hiredate;
}
public void setHiredate(Date hiredate) {
this.hiredate = hiredate;
}
public int getSal() {
return sal;
}
public void setSal(int sal) {
this.sal = sal;
}
public int getDeptno() {
return deptno;
}
public void setDeptno(int deptno) {
this.deptno = deptno;
}
public String getDname() {
return dname;
}
public void setDname(String dname) {
this.dname = dname;
}
public String getLoc() {
return loc;
}
public void setLoc(String loc) {
this.loc = loc;
}
}
|
cs |
EmpMapper (interface)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
package com.sist.dao;
import org.apache.ibatis.annotations.Select;
import java.util.*;
/*
* SubQuery : SQL문장을 하나로 묶어서 사용 => JOIN, 복잡한 쿼리 문장이 있는 경우, SQL문장이 많이 수행..
* = 종류
* 1. 단일행 서브쿼리
* 2. 다중행 서브쿼리
* ================= 조건문 사용 (WHERE ~)
* 3. 스칼라 서브쿼리
* ================= 컬럼 대체
* 4. 인라인 뷰(Top-N)
* ================= 테이블 대체
* = 실행
* 1. 서브쿼리가 실행 ==> 결과값 메인쿼리 전송
* 예) SELECT ~~
* FROM table_name
* WHERE 컬럼명 연산자 (SELECT ~~) ==> 서브쿼리는 먼저 수행 => 반드시 ()
* ==========
* 결과 1
* 결과 여러개 (IN, ANY, ALL)
* SELECT deptno FROM dept
* >ANY => 최소값 ANY(SELECT deptno FROM dept)
* ANY(10,20,30,40) => 10
* <ANY => 최대값
* ANY(10,20,30,40) => 40
* >ALL => 최대값 ALL(SELECT deptno FROM dept)
* ALL(10,20,30,40) => 40
* <ALL => 최소값
* ALL(10,20,30,40) => 10
* =============================================
* SELECT MIN(deptno) FROM dept
* SELECT MAX(deptno) FROM dept
* IN(10,20,30,40)
*
*/
public interface EmpMapper {
@Select("SELECT empno,ename,job,hiredate,sal,e.deptno,"
+"(SELECT dname FROM dept d WHERE e.deptno=d.deptno) as dname,"
+"(SELECT loc FROM dept d WHERE e.deptno=d.deptno) as loc "
+"FROM emp e")
public List<EmpVO> empListData();
@Select("SELECT empno,ename,job,hiredate,sal,deptno "
+"FROM emp "
+"WHERE deptno=(SELECT deptno FROM emp WHERE ename=#{ename})")
public List<EmpVO> empGroupData(String ename);
}
|
cs |
EmpDAO
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
package com.sist.dao;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
@Repository
public class EmpDAO {
@Autowired
private EmpMapper mapper;
public List<EmpVO> empListData(){
return mapper.empListData();
}
public List<EmpVO> empGroupData(String ename){
return mapper.empGroupData(ename);
}
}
|
cs |
com.sist.web
EmpController
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
package com.sist.web;
import java.util.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import com.sist.dao.*;
// Model 처리
/*
* <context:component-scan base-package="com.sist.*"/>
* ===============
* class를 찾는다
* @Controller @Repository @Service @RestController @Component
*/
@Controller
public class EmpController {
@Autowired
private EmpDAO dao;
@RequestMapping("emp/list.do")
public String emp_list(Model model){
List<EmpVO> list=dao.empListData();
model.addAttribute("list",list); // request.setAttribute("list", list)
return "list";
}
}
|
cs |
src/main/webapp/WEB-INF
web.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/config/application-*.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<!-- 한글 변환 -->
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<!-- /의 형식으로 시작하는 url에 대하여 UTF-8로 인코딩 -->
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
|
cs |
src/main/webapp/WEB-INF/config/
application-context.xml (Bean으로 생성 aop, beans, context, p, tx, util)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
|
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.3.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.3.xsd">
<!-- 어노테이션이 있는 클래스 메모리 할당 -->
<context:component-scan base-package="com.sist.*"/>
<!-- 데이터베이스 정보를 마이바티스로 전송
1. 정보를 모아서 처리 => BasicDataSource
데이터베이스 : 폴더
테이블 : 파일
-->
<bean id="ds" class="org.apache.commons.dbcp.BasicDataSource"
p:driverClassName="oracle.jdbc.driver.OracleDriver"
p:url="jdbc:oracle:thin:localhost:1521:XE"
p:username="hr"
p:password="happy"
/>
<!--
1. 오라클 : XE
2. Java => openjdk 15
3. STS => 3.9.14 => 스프링 5 (STS 4: Spring Boot)
-->
<bean id="ssf"
class="org.mybatis.spring.SqlSessionFactoryBean"
p:dataSource-ref="ds"
/>
<bean id="mapper"
class="org.mybatis.spring.mapper.MapperFactoryBean"
p:mapperInterface="com.sist.dao.EmpMapper"
p:sqlSessionFactory-ref="ssf"
/>
<!-- ViewResolver : JSP를 찾아서 request를 전송 -->
<!--
@Controller
public class MainController{
@RequestMapping("main/main.do")
public String main_main(){
=
=
=
return "main"; "board/list" "../member/join"
}
}
View Resolver
(prefix)main/main(suffix)
/main/main.jsp
-->
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:prefix="/emp/"
p:suffix=".jsp"
/>
<!--
Dispatcherservlet <=====> WebApplicationContext(Container)
= HandlerMapping : 클래스 찾기
= ViewResolver : JSP 찾기
= 사용자 정의 Controller
-->
</beans>
|
cs |
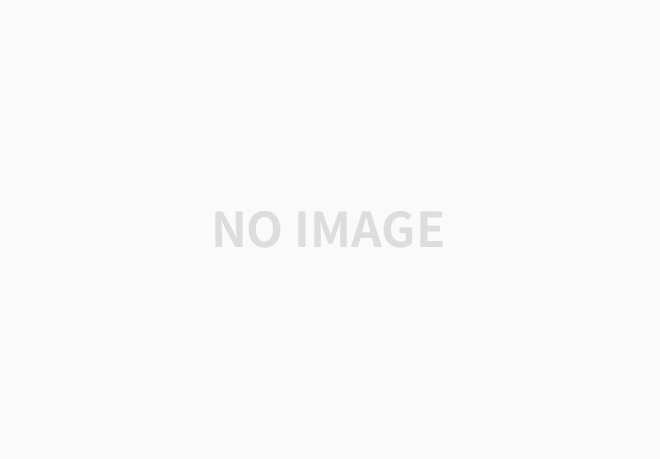
src/main/webapp/emp
list.jsp (React)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<style type="text/css">
.row{
margin: 0px auto;
width:900px;
}
</style>
<script src="https://unpkg.com/react@15/dist/react.js"></script>
<script src="https://unpkg.com/react-dom@15/dist/react-dom.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.34/browser.js"></script>
</head>
<body>
<div class="container">
<div class="row" id="root">
</div>
</div>
<!--
ES 5.0 text/javascript
ES 6.0 text/babel ==> react (ES6) ==> jsx (javascript+XML)
=====
1. 대소문자 구분
2. 여는 태그, 닫는 태그 동일
3. 태그를 사용할 경우 => 소문자 시작
React ==> Redux
(MVC) ==> saga, mobx(배달의 민족)
-->
<script type="text/babel">
class Emp extends React.Component{
render() {
return (
<table className="table table-striped">
<thead>
<tr className="success">
<th>사번</th>
<th>이름</th>
<th>직위</th>
<th>입사일</th>
<th>부서명</th>
<th>근무지</th>
</tr>
</thead>
<tbody>
<c:forEach var="vo" items="${list}">
<tr>
<td>${vo.empno}</td>
<td>${vo.ename}</td>
<td>${vo.job}</td>
<td><fmt:formatDate value="${vo.hiredate}" pattern="yyyy-MM-dd" /></td>
<td>${vo.dname}</td>
<td>${vo.loc}</td>
</tr>
</c:forEach>
</tbody>
</table>
)
}
}
ReactDOM.render(<Emp/>,document.getElementById('root'))
</script>
</body>
</html>
|
cs |
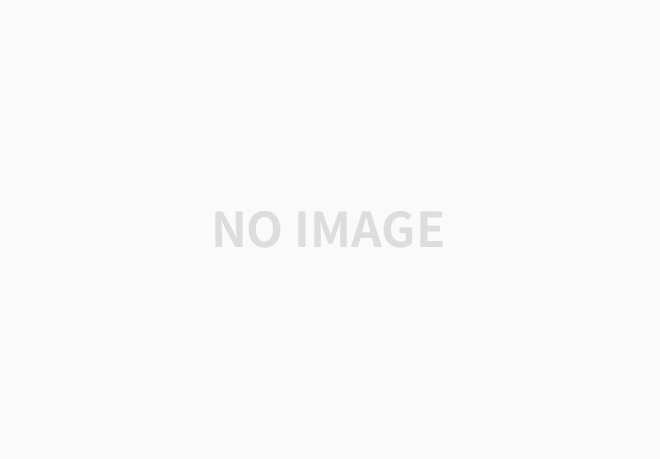
list2.jsp (jquery)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<style type="text/css">
.row{
margin: 0px auto;
width:900px;
}
</style>
<script type="text/javascript" src="http://code.jquery.com/jquery.js"></script>
<script type="text/javascript">
$(function(){
$('.names').hover(function(){
$(this).css("cursor","pointer");
},function(){
$(this).css("cursor","");
});
$('.names').click(function(){
let name=$(this).text(); // 클릭한 것을 가져오는 게 this
$.ajax({
type:'POST',
url:'sublist.do',
data:{"ename":name},
success:function(result){
$('#print').html(result);
}
});
})
});
</script>
</head>
<body>
<div class="container">
<div class="row">
<h1 class="text-center">사원 목록</h1>
<table class="table table-striped">
<tr class="success">
<th>사번</th>
<th>이름</th>
<th>직위</th>
<th>입사일</th>
<th>부서명</th>
<th>근무지</th>
</tr>
<c:forEach var="vo" items="${list}">
<tr>
<td>${vo.empno}</td>
<td><span class="names">${vo.ename}</span></td>
<td>${vo.job}</td>
<td><fmt:formatDate value="${vo.hiredate}" pattern="yyyy-MM-dd" /></td>
<td>${vo.dname}</td>
<td>${vo.loc}</td>
</tr>
</c:forEach>
</table>
</div>
<div class="row">
<h3 class="text-center">같은 부서에서 근무하는 사원 목록</h3>
<div id="print"></div>
</div>
</div>
</body>
</html>
|
cs |
EmpController
@RequestMapping과 return값을 list->list2로 해줘야 실행가능
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
package com.sist.web;
import java.util.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import com.sist.dao.*;
// Model 처리
/*
* <context:component-scan base-package="com.sist.*"/>
* ===============
* class를 찾는다
* @Controller @Repository @Service @RestController @Component
*/
@Controller
public class EmpController {
@Autowired
private EmpDAO dao;
@RequestMapping("emp/list2.do")
public String emp_list(Model model){
List<EmpVO> list=dao.empListData();
model.addAttribute("list",list); // request.setAttribute("list", list)
return "list2";
}
@RequestMapping("emp/sublist.do")
public String emp_sublist(String ename,Model model){
if(ename==null)
ename="KIN";
List<EmpVO> list=dao.empGroupData(ename);
model.addAttribute("list",list);
return "sublist";
}
}
|
cs |
sublist.jsp
화면변경없이 ajax를 통해 다른 jsp를 불러옴
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<style type="text/css">
.row{
margin: 0px auto;
width:900px;
}
</style>
</head>
<body>
<table class="table table-striped">
<tr class="success">
<th>사번</th>
<th>이름</th>
<th>직위</th>
<th>입사일</th>
<th>부서명</th>
<th>근무지</th>
</tr>
<c:forEach var="vo" items="${list}">
<tr>
<td>${vo.empno}</td>
<td>${vo.ename}</td>
<td>${vo.job}</td>
<td><fmt:formatDate value="${vo.hiredate}" pattern="yyyy-MM-dd" /></td>
<td>${vo.dname}</td>
<td>${vo.loc}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
|
cs |
반응형
'Back-end > Spring' 카테고리의 다른 글
Spring 13일차 - Mybatis연동, JSON (0) | 2020.11.13 |
---|---|
Spring 12일차 - PL/SQL Procedure 게시판 만들기 (0) | 2020.11.12 |
Spring 10일차 - 스프링 MVC (2) | 2020.11.09 |
Spring 8일차 - MyBatis 연동 (0) | 2020.11.03 |
Spring 7일차 - XML MVC구조 (0) | 2020.11.02 |