일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 이안의평일코딩
- 타입스크립트
- VUE
- typescript
- CSS
- 오라클
- Oracle
- 자바의정석
- 정보처리기사실기
- react
- 평일코딩
- 정보처리기사실기요약
- 국비IT
- php
- 리액트
- 정보처리기사실기정리
- 정보처리기사
- 코딩테스트
- 국비코딩
- spring
- 자스코테
- 정보처리기사정리
- javascript
- 자바스크립트 코딩테스트
- 정보처리기사요약
- 리액트네이티브
- Java의정석
- 스프링
- ReactNative
- 자바스크립트
- Today
- Total
이안의 평일코딩
JAVA의 정석 8일차 - 다중조건문 & 선택문 본문
2020.06.24(수)
1. 조건문
1)단일 조건문 if
2)선택 조건문 if~else
3)다중 조건문 if~else if~else if~else if~else
다중 조건문 => 조건이 많은 경우에 사용
형식)
if(조건식){
조건이 true면 수행하는 문장
수행후에 종료
}
else if(조건식){
조건이 true면 수행하는 문장
수행후에 종료
}
else if(조건식){
조건이 true면 수행하는 문장
수행후에 종료
}
else if(조건식){
조건이 true면 수행하는 문장
수행후에 종료
}
===================
else{
해당 조건이 없는 경우에 수행하는 문장
}
=================== 생략가능
======== 조건이 맞는 문장만 실행(1번만 수행)
int a = 15;
if(a%3==0)
System.out.println("3의 배수"); ==> 종료
else if(a%5==0)
System.out.println("5의 배수");
else if(a%7==0)
System.out.println("7의 배수");
===================================
차이) 단일 조건문 if => 독립적
if(a%3==0)
System.out.println("3의 배수"); ==> 종료
if(a%5==0)
System.out.println("5의 배수") ==> 종료
if(a%7==0)
System.out.println("7의 배수")
* 1) 데이터 저장
(1) 사용자가 요청 => 주로 사용
(2) File읽기
(3) 오라클 연결
(4) 웹에 있는 데이터 읽기
2) 사용자 요청한 내용으로 => 데이터 가공
3) 사용자에게 가공된 데이터를 출력
가공 => 연산자 / 제어문 => 메소드
* Scanner scan = new Scanner(System.in);
정수 => scan.nextInt()
문자열 => scan.next(), scan.nextLine()
실수 => scan.nextDouble()
논리 -> scan.nextBoolean()
문자 -> scan.next().charAt(0)
* 문자를 입력을 받아서 char ch
= 숫자 ch>='0' && ch<='9'
= 알파벳 ch>='A' && ch<='Z' || ch>='a' && ch<='z'
= 한글 ch>='가' && ch<='힣'
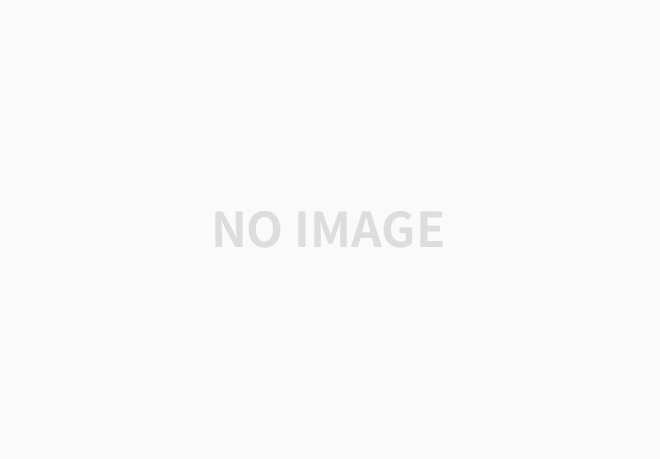
2. 선택문 switch
=> switch(정수,문자,문자열){
// 정수
case 정수값: => :
처리문장
==> if(조건){처리}
case 정수값:
처리문장 ===> 중복된 값은 사용할 수 없다
}
switch(a){
case 1:
== 라벨 (중복이 없다)
case 1: ==> error
if(a==1){System.out.println(1);}
if(a==1){System.out.println("일");}
if(a==1){
System.out.println(1);
System.out.println("일");
}
}
// 사용자 => 정수=1
switch(정수) ==> break가 없는 경우에는 모든 문장을 수행
{
case 1:
처리문장 1
case 2:
처리문장 2
break;
case 3:
처리문장 3
}
* case 정수 => case 1:
case 문자 => case 'A':
case 문자열 => case "+": case "LOGIN" "CHAT"...
===========================
int select=0;
Scanner scan = new Scanner(System.in);
System.out.print("정수(1~5):");
select=scan.nextInt();
// if(select==1) || select==2)
// 종료 시점 ==> break;
switch(select) {
case 1:
//System.out.println("1번~2번을 입력하였습니다");
//break; //switch문 종료한다
case 2:
System.out.println("1번~2번을 입력하였습니다");
break;
case 3:
System.out.println("3번을 입력하였습니다");
break;
case 4:
System.out.println("4번을 입력하였습니다");
break;
case 5:
System.out.println("5번을 입력하였습니다");
break;
default:
System.out.println("1~5번까지만 사용하세요");
}
===========================
3. 중첩
if(조건문){
if(조건문){
}
else{
}
}
switch(정수){
case 1:
switch(정수){
case 'A':
}
}
4. 지역변수
public static void main(String[] args) {
int a = 10;
switch(a) {
case 10 : {
int b = 100;
System.out.println("a="+a+",b="+b);
break;
}
case 11 : {
int b = 200;
System.out.println("a="+a+",b="+b);
break;
}
}
}
=> {} 블럭 설정을 하면 블럭 안에서 지역변수가 되어
변수 b가 이름변경 없이 다른 블럭에서 재사용 가능해짐
5. 연습문제
1) 성적 계산
==> 3개의 점수를 받아서 총점, 평균, 학점 => 등수
import java.util.*;
public class 성적계산 {
public static void main(String[] args) {
//변수 => 저장공간을 설정
int kor=0,eng=0,math=0;
int total=0;
double avg=0.0;
char hakjum='A';
//입력을 받는다 => 변수에 저장
Scanner scan = new Scanner(System.in);
System.out.print("국어 점수 입력:");
kor = scan.nextInt(); // 키보드로 입력한 정수를 읽어온다
System.out.print("영어 점수 입력:");
eng = scan.nextInt();
System.out.print("수학 점수 입력:");
math = scan.nextInt();
// 1. 총점
total = kor + eng + math;
// 2. 평균
avg = total / 3.0; // 소수점
// 3. 학점
if (avg>=90)
hakjum='A';
else if (avg>=80)
hakjum='B';
else if (avg>=70)
hakjum='C';
else if (avg>=60)
hakjum='D';
else // 50, 40, 30, 20, 10
hakjum='F';
// 4. 처리가 완료 => 화면에 출력
System.out.println("====== 성적 ======");
System.out.println("국어 점수:"+kor);
System.out.println("영어 점수:"+eng);
System.out.println("수학 점수:"+math);
System.out.println("총점:"+total);
System.out.printf("평균:%.2f\n",avg);
System.out.println("학점:"+hakjum);
}
}
2) 각 달의 마지막 날짜 출력
31, 28, 31, 30, 31, 30
31, 31, 30, 31, 30, 31
=> 28, 30, 31
import java.util.*;
public class 마지막날짜 {
public static void main(String[] args) {
int month=0;
Scanner scan = new Scanner(System.in);
System.out.print("원하는 월을 입력(1~12):");
month = scan.nextInt();
//메모리에 저장 => 변수
if(month==4 || month==6 || month==9 || month==11){
System.out.println(month+"월은 30일까지 있습니다");
}
else if(month==2){
System.out.println(month+"월은 28일까지 있습니다");
}
else{
System.out.println(month+"월은 31일까지 있습니다");
}
}
}
3-1) 사칙연산 (다중 조건문 if~else if~else if~else)
변수 => 정수 2개, 연산자(+,-,*,/)
import java.util.*;
public class 사칙연산 {
public static void main(String[] args){
//변수
//정수 2개
int a=0, b=0;
//char 1개
char op='+';
//사용자의 입력값을 받는다
Scanner scan = new Scanner(System.in);
System.out.print("두개의 정수 입력(90 100):");
a=scan.nextInt();
b=scan.nextInt();
System.out.print("연산자 입력(+,-,*,/):");
op=scan.next().charAt(0);
if(op=='+'){
System.out.printf("%d+%d=%d\n",a,b,a+b);
}
else if(op=='-'){
System.out.printf("%d-%d=%d\n",a,b,a-b);
}
else if(op=='*'){
System.out.printf("%d*%d=%d\n",a,b,a*b);
}
else if(op=='/'){
if(b==0){
System.out.println("0으로 나눌 수 없습니다");
}
else{
System.out.printf("%d/%d=%.2f\n",a,b,a/(double)b);
}
}
else{
//연산자외에 다른 문자를 입력 했을 때 처리
System.out.println("잘못된 연산자 입력입니다!!");
}
}
}
3-2) 사칙연산 (선택문 switch case break)
import java.util.*;
public class 사칙연산2 {
public static void main(String[] args) {
int a=0, b=0;
int result=0;
Scanner scan = new Scanner(System.in);
System.out.print("정수 2개 입력>");
a=scan.nextInt();
b=scan.nextInt();
System.out.print("연산자 입력(+,-,*,/):");
//String op=;
switch(scan.next()) {
case "+" :
System.out.printf("%d+%d=%d\n",a,b,a+b);
break;
case "-" :
System.out.printf("%d-%d=%d\n",a,b,a-b);
break;
case "*" :
System.out.printf("%d*%d=%d\n",a,b,a*b);
break;
case "/" :
if(b==0) {
System.out.println("0으로 나눌 수 없습니다!!");
}
else {
System.out.printf("%d/%d=%d\n",a,b,a/(double)b);
}
break;
default:
System.out.println("잘못된 연산자 입니다!!");
}
}
}
4) 웹사이트 연결
import java.util.*;
public class 웹연결 {
public static void main(String[] args) throws Exception{
int menu=0;
Scanner scan = new Scanner(System.in);
System.out.println(
"===== 메뉴 =====\n"
+"홈(1)\n현재상영영화(2)\n개봉예정영화(3)\n박스오피스(4)\n"
+"영화예매(5)\n영화뉴스(6)\n"
+"=============="
);
System.out.println("메뉴를 선택하세요>");
menu=scan.nextInt();
switch(menu) {
case 1:
Runtime.getRuntime().exec("C:\\Program Files\\Internet Explorer\\iexplore.exe https://movie.daum.net/main/new#slide-2-0");
break;
...........
case 6:
...........
break;
default:
System.out.println("메뉴가 존재하지 않습니다");
}
}
}
5) 학점 계산
3개의 정수를 입력받아서 학점 계산
import java.util.*;
public class 학점계산 {
public static void main(String[] args) {
int kor=0, eng=0, math=0;
char score='A';
// ==> 프로그램에 필요한 메모리 저장 공간 확보
// 입력
Scanner scan = new Scanner(System.in);
System.out.print("점수 3개를 입력하세요");
kor=scan.nextInt();
eng=scan.nextInt();
math=scan.nextInt();
// 결과값을 출력 => 가공 (연산자+제어문)
// 메소드 => 데이터를 가공해서 결과값을 넘겨준다
switch((kor+eng+math)/30) {
case 10:
case 9:
score='A';
break;
case 8:
score='B';
break;
case 7:
score='C';
break;
case 6:
score='D';
break;
//case 5: ..... case 1:
default:
score='F';
break;
}
System.out.println("학점 :"+score);
}
}
6-1) 가위바위보 (선택문 switch, case, break)
import java.util.*;
public class 가위바위보 {
public static void main(String[] args) {
int user=0;
int com=(int)(Math.random()*3); // 0, 1, 2
Scanner scan = new Scanner(System.in);
System.out.print("가위(0), 바위(1), 보(2):");
user=scan.nextInt();
switch (com) {
case 0:
switch(user) {
case 0:
System.out.println("컴퓨터:가위,사용자:가위");
System.out.println("비겼다!!");
break;
case 1:
System.out.println("컴퓨터:가위,사용자:바위");
System.out.println("사용자 Win!!");
break;
case 2:
System.out.println("컴퓨터:가위,사용자:보");
System.out.println("컴퓨터 Win!!");
break;
}
break;
case 1:
switch(user) {
case 0:
System.out.println("컴퓨터:바위,사용자:가위");
System.out.println("컴퓨터 Win!!");
break;
case 1:
System.out.println("컴퓨터:바위,사용자:바위");
System.out.println("비겼다!!");
break;
case 2:
System.out.println("컴퓨터:바위,사용자:보");
System.out.println("사용자 Win!!");
break;
}
break;
case 2:
switch(user) {
case 0:
System.out.println("컴퓨터:보,사용자:가위");
System.out.println("사용자 Win!!");
break;
case 1:
System.out.println("컴퓨터:보,사용자:바위");
System.out.println("컴퓨터 Win!!");
break;
case 2:
System.out.println("컴퓨터:보,사용자:보");
System.out.println("비겼다!!");
break;
}
break;
}
}
}
번외 6-2) 가위바위보 (String)
String[] str= {"가위","바위","보"};
System.out.println("컴퓨터:"+str[com]
+",사용자:"+str[user]);
switch(com-user) {
case 2: case -1:
System.out.println("사용자 Win!!");
case 1: case -2:
System.out.println("컴퓨터 Win!!");
case 0:
System.out.println("비겼다!!");
}
'Back-end > Java' 카테고리의 다른 글
JAVA의 정석 10일차 - 반복문 while (0) | 2020.06.26 |
---|---|
JAVA의 정석 9일차 - 반복문 for (0) | 2020.06.25 |
JAVA의 정석 7일차 - 연산자정리 & 조건문2 (0) | 2020.06.23 |
JAVA의 정석 6일차 - 조건문 (0) | 2020.06.22 |
JAVA의 정석 5일차 - 연산자2 (0) | 2020.06.22 |